Published by
Many in the API community are aware of the concept of “API First”, but most articles I see on the subject simply describe what API First is and some of its benefits. Of course, learning about API First and convincing your colleagues to adopt an API First approach to developing features and applications is half the battle, but what does the actual implementation look like? In this post, we’ll walk through the details of how you might implement an API Design-First approach, which is one of the many flavors of the broad API First concept.
API Design-First — A Quick Refresher
In short, API Design-First is a way of developing application features where the design and shape of the API is considered before any frontend or backend development actually begins. A top-down approach is typically followed where the Experience Design (XD) drives the needs and capabilities of the API. By iterating on the contract and interactions between the frontend and backend, both teams get a chance to become familiar with what the API will look like before implementation, which leads to a smooth and natural integration process. For more information on API First, API Design-First, and its many other variations, check out these awesome articles by some of the top API platforms:
- Swagger — Understanding the API-First Approach to Building Products
- Stoplight — API-First, API Design-First, or Code-First: Which Should You Choose
- Postman — Guide to API-First
Process Overview
Like much of the typical development lifecycle, API Design-First follows a “design and build” process to produce iterations of features. At a high-level, the process looks something like the following:

XD Artifact Handoff
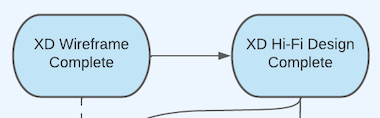
The process begins with some sort of handoff of an XD artifact, whether that be a wireframe or hi-fi screen design of some other aspect of a user experience flow or feature. While waiting for an XD artifact to be produced and finalized is not strictly necessary, we find that following a top-down approach generally leads to a better developer and user experience by enabling the powerful concept of Experience APIs.
API Domain Definition
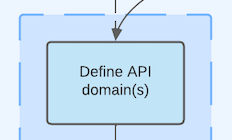
Armed with the XD artifact, the core API design process begins with defining the underlying API domain(s) necessary to support the feature. This might already exist, in the case of something like an existing microservice, or it’s possible that an entirely new API concept might need to be created. For instance, while making your way through the design process for an eCommerce application, you might end up defining API domains like “Account”, “Categories”, “Products”, “Ordering”, etc.
API Endpoint Definition
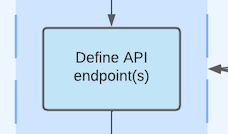
Once you’ve established the proper API domain for a feature to live, you can begin outlining the endpoints that will be necessary to bring that feature to life. In terms of a REST API, this would be the resources that make up your API. It’s important to keep things high-level at this stage so just describing the HTTP method and endpoint name/structure are all that’s necessary. For instance, continuing with the eCommerce application example, some endpoints you might define for the “Products” API might be things like GET /products?category={category}
to retrieve all of the products for a given category and GET /products/{productId}
to get the details for a specific product.
Request/Response Definition

After establishing an API domain and its basic resources and actions, you can start to iron out the details of the request and response structures for each endpoint. The reason we’ve waited until now to start putting these details together is because API contract design can sometimes be an iterative process in the early stages of a new feature or product. You want to give yourself room to explore various combinations of API domains and shapes before spending time doing the majority of the work to describe and document the API.
In addition to things like the JSON schemas used in the requests and responses, you’ll also want to take the time to come up with helpful descriptions for other API components like endpoints, schemas, headers, etc. If necessary, you can also create supporting documentation pages around concepts like authentication, pagination, caching, or even a domain-specific concept like details about the “cart” functionality in the case of the eCommerce example.
While it’s possible to go low-tech here by simply describing the API details in something like a Word or markdown document, it is advisable to use some sort of machine-readable specification format like OpenAPI (formally known as Swagger). Doing so allows you to take advantage of modern tooling that will make it much easier to create consistent API contracts as well as do things like automatically generate documentation pages, mock servers, and helper libraries.
Request/Response Mapping
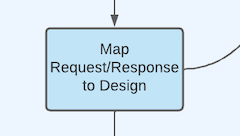
Once the details of an API are in place, you’re ready to begin coding, right? Wrong! Just as developing quality software is enabled by writing unit tests, writing quality API contracts is enabled by performing some sort of mapping exercise. Doing so gives you an opportunity to quickly “test” your specification and check your work before handing it off to development teams to implement. A basic mapping document might look something like the following, where you put the XD screen designs side-by-side with the details of the endpoint(s) that will power that feature:

While you do sort of go through this process in your head when initially describing the API, doing it “on paper” really puts the API design to the test. The main idea is to try to find as many gaps, inconsistencies, or awkwardness in the intended usage of the API. Of course, you could also write code that exercises the API against a mock service, but it’s not strictly necessary unless you want to go the extra mile to test or prototype things out before publishing.
API Specification Versioning & Publishing

Once an API concept has been fully explored and the details defined, it’s time to introduce or increment the version of the API contract. Implementing good versioning practices, such as semantic versioning, is critical to ensuring that consumers of the API are not caught by surprise when breaking changes are made to the spec. This is just as much true early on in the development process as it is when the API has already been released to production. Early in the lifecycle of an API, development teams may be consuming pre-release versions of an API that are rapidly changing and evolving as requirements are finalized and iterated upon. Building some level of trust that breaking changes in the API can be easily identified and planned for will lead to much happier and productive development teams.
Once an API contract has been properly versioned, it can then be published and made available for others to view. This may happen in some sort of automated way, such as a system parsing an OpenAPI document and auto-generating the corresponding HTML pages to render the documentation. It may also be as simple as uploading the document to a file server or emailing it to colleagues.
API Specification Review & Acceptance

Once an initial version or update to an API design has been published, it’s critical to solicit feedback from other stakeholders involved in the creation or consumption of an API. While doing things like the mapping exercise is meant to reduce the amount of potential rework that might be necessary, there’s always the possibility that you forgot to consider a certain scenario or that stakeholders just don’t like the way something is designed. This may necessitate an iteration on the API contract.
Incorporating feedback might be as simple as renaming some endpoints or properties, or it might be more involved. For complex features, it’s possible that you may end up needing to go back and rethink the domains or reorganize shapes of the API. Naturally, any updates made to the contract should then run through the remaining steps of the API design process. At a minimum, updated mapping documents and associated documentation should be produced, along with a stakeholder re-review of the now updated API designs. Once all feedback has been addressed, the API contract can be considered “accepted” and coding can begin.
Build Handoff

Once an API has been identified, described, and its interface agreed upon, both frontend and backend development can begin in parallel. By utilizing mocking techniques, frontend developers can reliably develop features without a working backend. Backend developers should also have a clear understanding of the API contracts that they need to fulfill. When the backend code comes online, things should “just work” for the frontend. While often times, this can be more a dream than a reality, it is made much easier to achieve by having a precise API specification that can be used to test and verify the behavior of the implemented API.
Conclusion
I hope this article helps to bring some clarity as to what an API Design-First process might look like for your team. Even if you don’t follow these steps exactly, the end result should be the same. API Design-First enables a smooth development experience by carefully considering the needs of the user as well as incorporating feedback from development teams early and often. All of this happens before coding begins, which helps to minimize the cost associated with changes to functionality or clarification of requirements.
In a future post, we’ll take a look at some essential tooling that enables a good API Design-First experience. Until then, what do you think? Have you implemented an API Design-First process for your team or company? What have you found that works or doesn’t work? I’d love to hear your thoughts! Feel free to comment below or contact me at [email protected] to chat about all things API First.